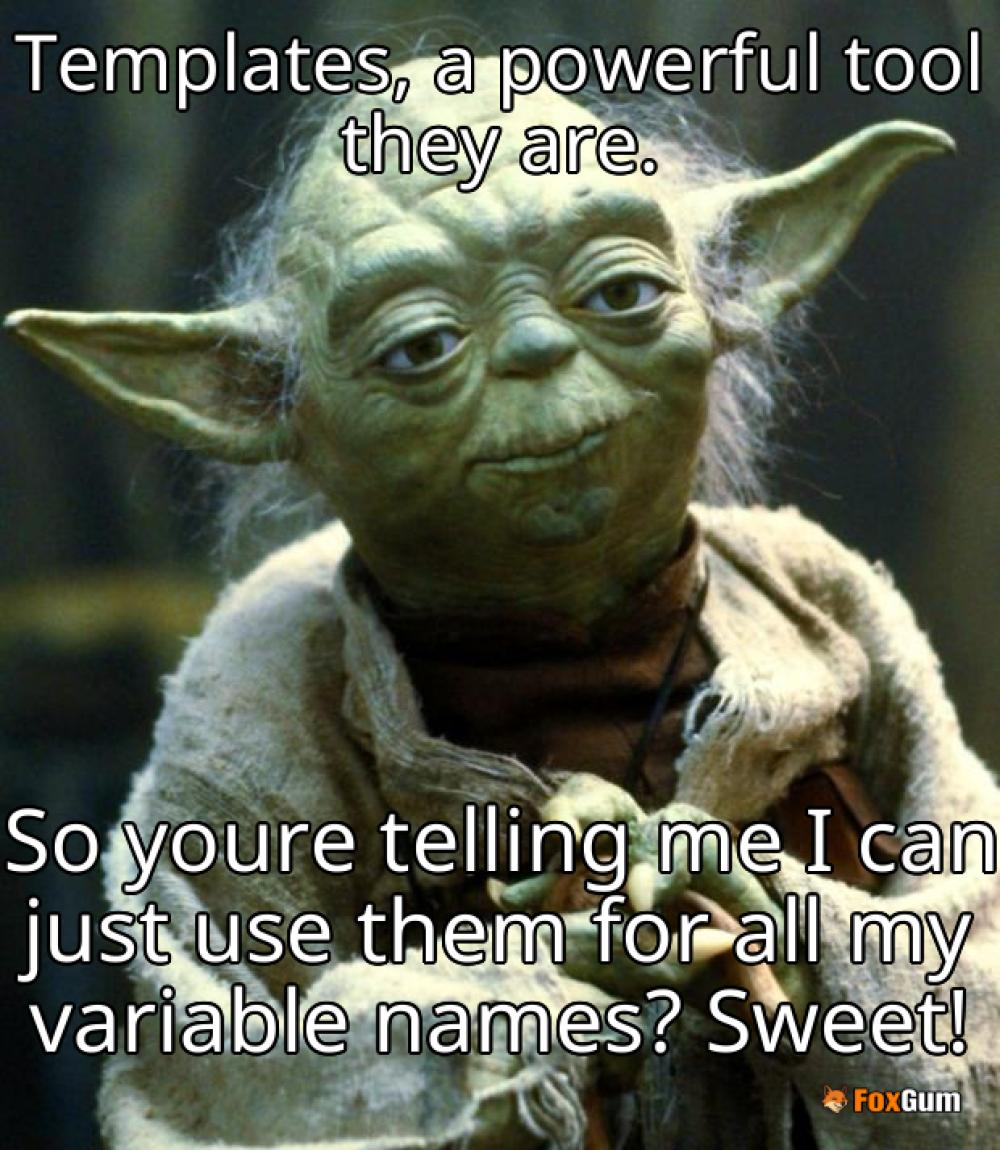
Unleashing the Power of Templates in C++ ๐
Welcome to the wild world of C++ templates, where coding meets creativity! If you’re tired of writing the same old functions over and over again for different data types, templates are about to become your new best friend. Imagine being able to whip up a function that works for integers, floats, and even strings without breaking a sweat! Let’s dive into this coding wonderland and see what templates are all about.
What Are Templates Anyway? 🤔
In simple terms, templates in C++ allow programmers to create functions and classes that can operate with any data type. Instead of creating multiple versions of the same function for different types, you can write one function and let the magic of templates do the heavy lifting! 🎩✨
Types of Templates
- Function Templates: These allow you to create a generic function. For example, a function to sort an array can be written once and used for any data type.
- Class Templates: Similar to function templates, but for classes! You can define a class that can handle any data type. Think of it as a superhero class that saves the day for all data types! 🦸♂️
How to Create a Template in C++
Creating a template is as easy as pie! 🥧 Here’s the basic syntax:
template <typename T>
T myFunction(T param) {
return param;
}
Just replace T with whatever name you want to give to your data type! Easy peasy, right?
Benefits of Using Templates
Why should you care about templates? Here are a few reasons:
- Code Reusability: Write once, use everywhere! Templates save you from the repetitive grind of coding.
- Type Safety: Templates ensure that you’re using the right data types, reducing the chances of errors. Less debugging, more coding! 🐞
- Performance: Templates are compiled at compile time, which can lead to faster execution. Who doesn’t want a speedy program? ⚡
Common Pitfalls to Avoid
Even though templates are pretty awesome, they’re not without their quirks. Here are some things to watch out for:
- Template Bloat: Too many templates can lead to larger binaries. Keep it tidy!
- Complex Error Messages: When templates go wrong, the error messages can be a real head-scratcher. Be prepared for some puzzling moments! 🧩
Final Thoughts 💭
Templates in C++ are like the Swiss Army knife of programming! They offer flexibility, efficiency, and a sprinkle of fun to your coding life. So, go ahead, give templates a whirl, and watch your code transform into a lean, mean, data-handling machine! Happy coding! 🎉